The beauty of developing the app in Flutter is that you can customize your app the way you want. You can change almost anything in Flutter. For example, you may want to customize the app that speaks your brand language. and you may want to start with changing the background color of your screen. So in this tutorial, we’ll learn the 2 easy ways to change background color of screen/scaffold in Flutter.
Here’s how it will look:
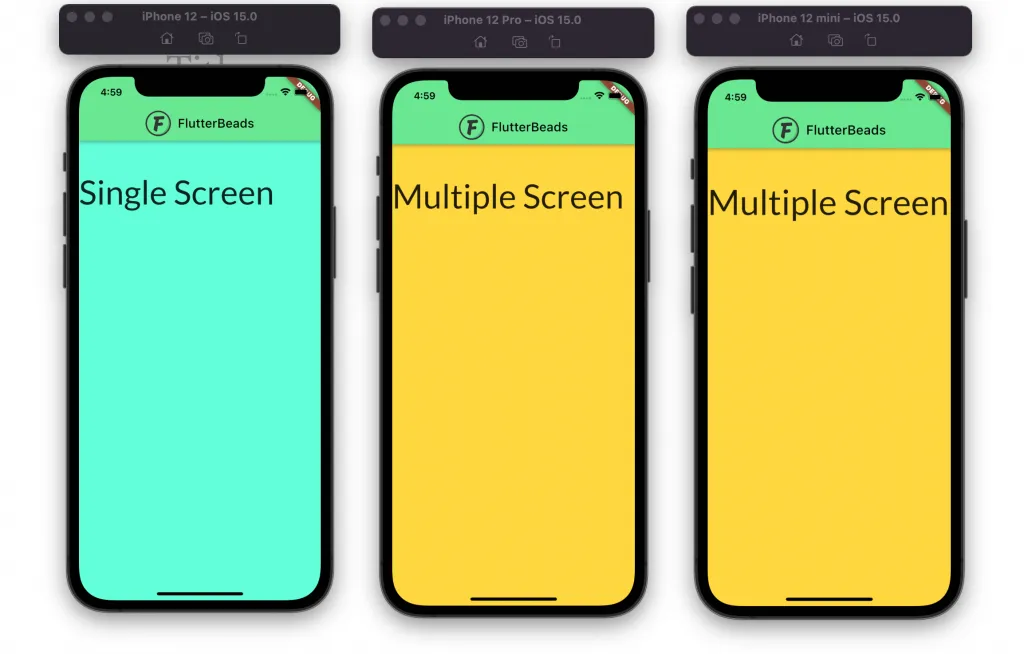
Here’s what we’ll cover:
The Problem
When you create a new app in Flutter, by default the background color is set to the Colors.grey[50]
which almost resembles the white color. And this color is set for all the new screens that you create with the Scaffold widget.

Ways to Change Background Color of Screen/Scaffold in Flutter
There are main two ways you can change background color of screen/scaffold in Flutter.
- Using Scaffold Widget
- Using ThemeData widget (Inside MaterialApp)
You can use any of these based on your requirement.
1. Using Scaffold Widget
To change the background color of screen using the Scaffold widget, just add the backgroundColor property and assign the color you want.
When to Use:
Use this approach when you want to change the background color only on a specific screen.
Steps:
Step 1: Make sure you have a Scaffold widget added.
Step 2: Inside the Scaffold widget, add the backgroundColor property and assign the color. (e.g. backgroundColor: Colors.amberAccent.)
Step 3: Run your app.
Code Example:
@override Widget build(BuildContext context) { return Scaffold( backgroundColor: Colors.amberAccent, //<-- SEE HERE appBar: ..., body: ..., ); }
Output:

2. Using ThemeData widget (Inside MaterialApp)
To change the background color of the screen using the ThemeData widget, just add the scaffoldBackgroundColor property and assign the color you want.
When to Use:
Use this approach when you want to change the background on all screens of your app.
Steps:
Step 1: Go to your main.dart file.
Step 2: Inside the MaterialApp, find the ThemeData widget.
Step 3: Add the scaffoldBackgroundColor property inside and assign the color you want. (e.g. scaffoldBackgroundColor: Colors.tealAccent).
Step 4: Restart the app.
Code Example:
@override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, scaffoldBackgroundColor: Colors.tealAccent, //<-- SEE HERE ), home: home(), ); }
Output:

Conclusion
In this tutorial, we learned how to change background color of screen/scaffold in Flutter with practical examples. Basically, we can change the background color using the Scaffold and ThemeData widget and saw when to use which approach.
Would you like to check other interesting Flutter tutorials?