Flutter allows you to develop a multi-platform app that runs on a mobile, web, and desktop from a single codebase. While developing a multi-platform app, you may want to design a UI or build a feature that works differently on all the platforms. To accomplish this, you must determine the platform. So in this article, we’ll learn how to check platform in Flutter.
Here’s what we’ll cover in this article:
- What is Platform
- The Necessity of Checking Platform
- How to Check Platform in Flutter
- Platforms Available to Check
- Check Top Level Platform
What is Platform
According to the definition on Wikipedia, a Platform is an environment in which the code is executed. A Platform can be hardware or software. In the Flutter world, operating systems and web browsers are considered to be the platform.
List of platforms supported by Flutter:
- Mobile: You can develop a mobile app for Android and iOS.
- Web: Build a SPA (Single page app)or PWA app for browsers such as Chrome, Mozilla Firefox, etc.
- Desktop: You can also develop an app for Windows, MAC, and Linux operating systems.
- Embedded: You can run Flutter apps on any new hardware and operating systems using a clean interface for custom embedders.
The Necessity of Checking Platform
Without checking the platform, your app mostly looks and works the same way on all the platforms. In case if you need to implement a feature slightly different on one of the platforms, you need to detect it in Flutter.
Some examples:
- You may want to layout material widgets in Android and Cupertino widgets in iOS.
- Disabling a few features on the web and continue to allow on mobile.
How to Check Platform in Flutter
The platform in Fluter is determined by checking the defaultTargetPlatform
and comparing it with TargetPlatform
enum.
Steps to check platform in Flutter:
Step 1: Add the import statementimport ‘package:flutter/foundation.dart’;
to your file.
Step 2: Add the following if statement in your code:
if (defaultTargetPlatform == TargetPlatform.android){ // YOUR CODE }
- The above code snippet checks if the current platform is Android or not.
- It first detects the current platform using defaultTargetPlatform and compares it with the values inside the TargetPlatform.
- Write a code inside the if statement that you want to perform only in Android.
Step 3: Similarly add check for other platforms that looks like the following:
if (defaultTargetPlatform == TargetPlatform.android){ // Android specific code } else if (defaultTargetPlatform == TargetPlatform.iOS){ //iOS specific code } else { //web or desktop specific code }
Step 4: Run the app.
Final Code
By applying the above steps for detecting the platform in Flutter, we can design a UI that shows the platform text on the respective platform.
Here’s the code:
Column( mainAxisAlignment: MainAxisAlignment.center, children: [ if (defaultTargetPlatform == TargetPlatform.android) ...[ const Center( child: Text( 'Android', style: TextStyle(fontSize: 74, fontWeight: FontWeight.bold), ), ) ] else if (defaultTargetPlatform == TargetPlatform.iOS) ...[ const Center( child: Text( 'iOS', style: TextStyle(fontSize: 74, fontWeight: FontWeight.bold), ), ) ] else ...[ const Center( child: Text( 'Web', style: TextStyle(fontSize: 74, fontWeight: FontWeight.bold), ), ) ] ], )
Preview:
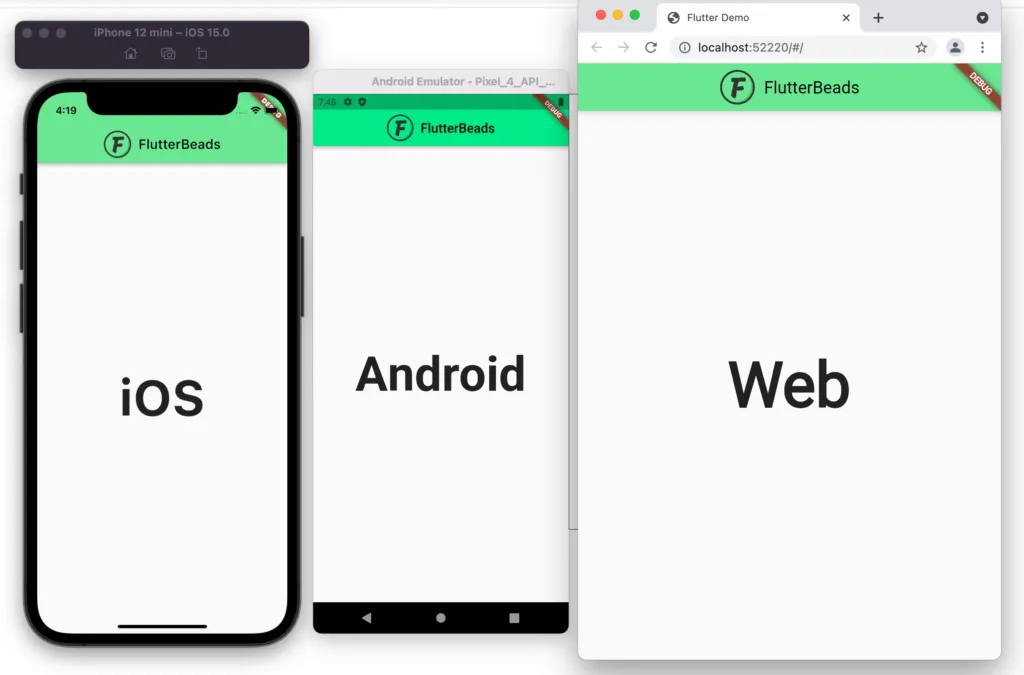
Platforms Available to Check
The TargetPlatform currently supports the following platforms:
enum TargetPlatform { android, fuchsia, iOS, linux, macOS, windows, }
Hint: If you see carefully, it doesn’t contain the web platform. To detect if the platform is a web, simply put web compatible code in your last else statement.
Check Top Level Platform
Sometimes you may need to layout or perform some operation based on the top-level platform such as mobile, desktop, and web.
Here’s how you detect the top-level platform:
- Create a file called platform_details.dart in your project.
- Add the following singleton class which combines the multiple platforms and prepares a top-level platform getter.
import 'package:flutter/foundation.dart'; class PlatformDetails { static final PlatformDetails _singleton = PlatformDetails._internal(); factory PlatformDetails() { return _singleton; } PlatformDetails._internal(); bool get isDesktop => defaultTargetPlatform == TargetPlatform.macOS || defaultTargetPlatform == TargetPlatform.linux || defaultTargetPlatform == TargetPlatform.windows; bool get isMobile => defaultTargetPlatform == TargetPlatform.iOS || defaultTargetPlatform == TargetPlatform.android; }
- Use the getters to determine the top-level platform like this:
Here’s the full code:
Column( mainAxisAlignment: MainAxisAlignment.center, children: [ if (PlatformDetails().isMobile) ...[ const Center( child: Text( 'Mobile', style: TextStyle(fontSize: 74, fontWeight: FontWeight.bold), ), ) ] else if (PlatformDetails().isDesktop) ...[ const Center( child: Text( 'Desktop', style: TextStyle(fontSize: 74, fontWeight: FontWeight.bold), ), ) ] else ...[ const Center( child: Text( 'Web', style: TextStyle(fontSize: 74, fontWeight: FontWeight.bold), ), ) ] ], )
Preview:
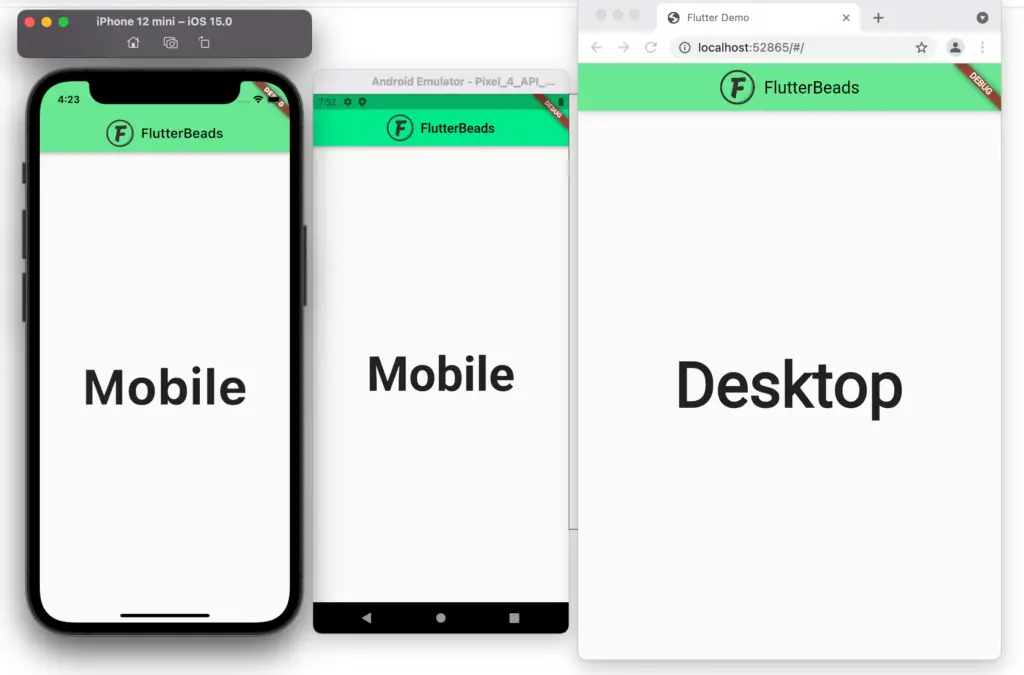
Conclusion
In this tutorial, we learned how to check platform in Flutter with a practical example. We also learned why need to perform such checks and also how to detect the top-level platform.
Take a look at other interesting Flutter tutorials.