While developing the Fluter app, you must be referring to a design file to create the UI for the app. Most of the design file allows you to copy the color in Hexadecimal (Hex) color code string. Even if you are taking colors from any website (Using google chrome inspect) it gives you the hex code for the color. so how do you use color from hex in Flutter? In this tutorial, we’ll learn the 2 ways to use the Hexadecimal (Hex) Color Code String in the Flutter.
Here’s what we’ll cover:
- What is the Hexadecimal Color Code String
- The problem
- How to use color from hex in Flutter
- Changing the Opacity
The Problem
The problem with the Hex color code in Flutter is that you can’t use it directly like this:
backgroundColor: Color('#6ae792'), // Gives error

The Color class in Flutter only accepts integers as parameters. So we need some way to use/convert the hex code #6ae792 into the integer format that the Color class can understand.
How to use color from hex in Flutter
There are two ways you can use flutter hex color:
Steps to use color from hex in Flutter:
- Remove the # sign.
- Add 0xFF at the beginning of the color code.
- Place it inside the Color class like this Color(0xFFbfeb91).
backgroundColor: Color(0xFFbfeb91), // Will work
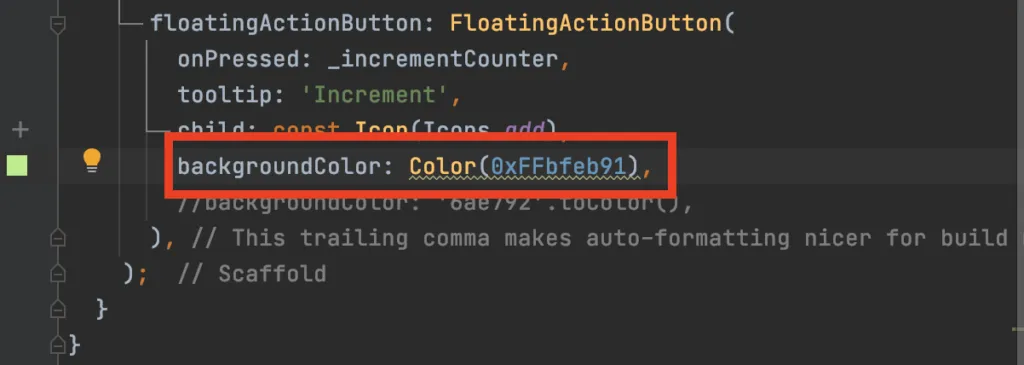
Steps to use Hexadecimal (Hex) Color Code using Extention
Step 1: Create a new file extentions.dart
under the lib folder and add the below code.
import 'package:flutter/material.dart'; extension ColorExtension on String { toColor() { var hexString = this; final buffer = StringBuffer(); if (hexString.length == 6 || hexString.length == 7) buffer.write('ff'); buffer.write(hexString.replaceFirst('#', '')); return Color(int.parse(buffer.toString(), radix: 16)); } }
The above code replaces the # with ff and converts a string into the integer value.
Step 2: To use the extension, simply add .toColor() at the end of the hex color like this ‘#bfeb91’.toColor().

Changing the Opacity
The FF or ff, in the beginning, indicates the color is opaque. Modifying this value will change the opacity of the color.
You can use any value from the below to change the opacity.
100% - FF 95% - F2 90% - E6 85% - D9 80% - CC 75% - BF 70% - B3 65% - A6 60% - 99 55% - 8C 50% - 80 45% - 73 40% - 66 35% - 59 30% - 4D 25% - 40 20% - 33 15% - 26 10% - 1A 5% - 0D 0% - 00
Here’s how it works:
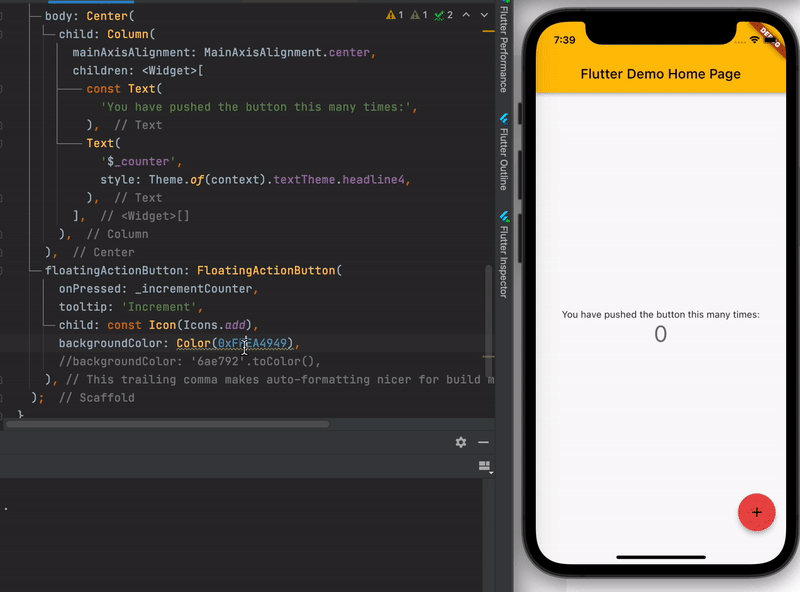
Conclusion
In this tutorial, we learned 2 Ways to Use color from hex in Flutter with additional knowledge on what is Hex color code and how to change the opacity.
FAQs
Can we use hex code in Flutter?
Yes, you can use hex code in Flutter. Hexadecimal color codes are a standard way to represent colors in web development, and Flutter supports using them as well.
In Flutter, you can use hexadecimal color codes by replacing the # with 0xFF. For example, if you want to use the color code #ff0000 (which represents red), you can use it like this: Color redColor = Color(0xFFFF0000);
More Articles: