Flutter allows you to combine various widgets to develop a UI that matches your design. While developing a screen in the Flutter app, you may add a Text widget inside a Row widget and you might have got the overflow error with a long text. This can be fixed by wrapping a text. So in this tutorial, we’ll learn how to avoid Flutter Text overflow with proven wrap strategies.
Here’ what well cover:
The Problem
While showing a dynamic response from an API, you may get a long text in response. When the text is sufficiently long enough that it won’t fit in the width of the screen, you will get an overflow error.
Here’s is how it looks:
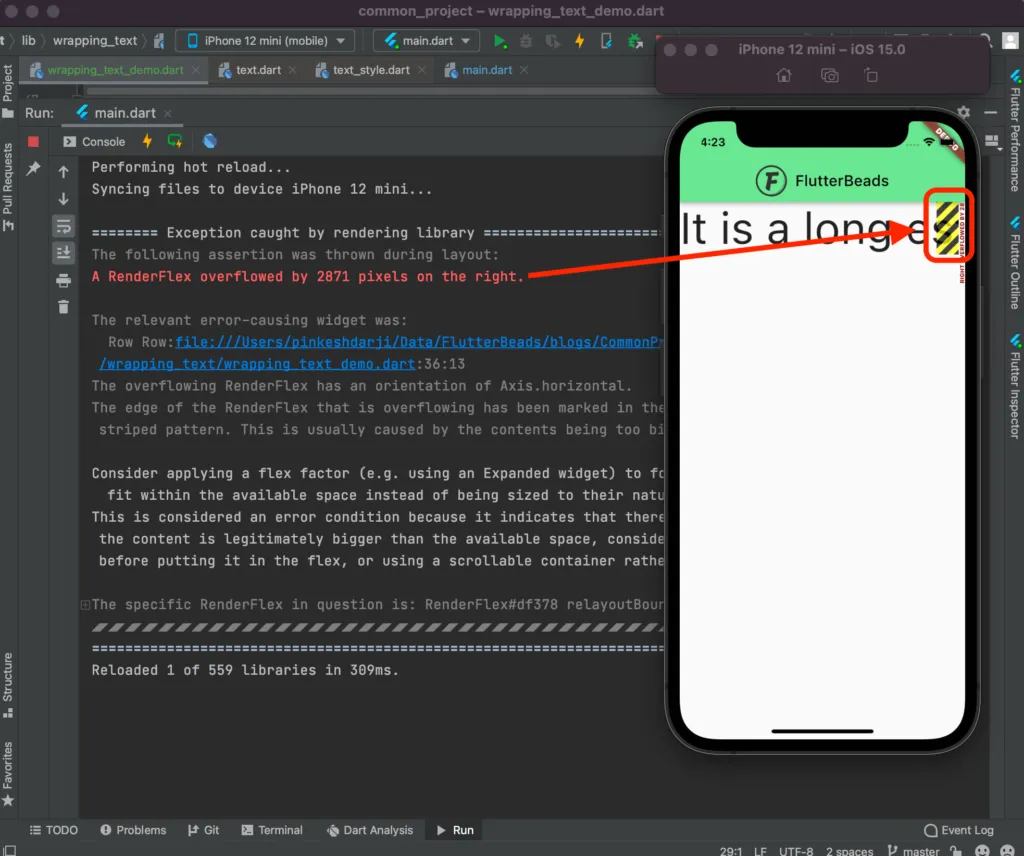
Steps to avoid Flutter Text overflow
Flutter text wrap is a technique used to avoid text overflow in a Flutter app by wrapping the Text widget inside an Expanded widget. The Expanded widget allows the Text widget to grow and fill the available space. When you wrap the Text widget (with long text) inside the Expanded widget, the text which can be visible in the screen’s width will be displayed and the rest of the text portion will be clipped off.
To avoid Flutter Text overflow:
- Make sure your Text widget is inside the Row widget.
- Wrap your Text widget inside the Expanded widget.
- Run your app.
Code:
Row( children: const [ Expanded( child: Text( 'It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout.', style: TextStyle(fontSize: 58), softWrap: false, maxLines: 1, ), ), ], )
Output:

Showing Ellipsis on Overflow
Showing the clipped text on the screen is not a good idea. You never know what meaning a half text will convey :) Instead, it’s better to give the user an idea about the continued text when it’s overflowing. The text continuity can be represented with the Ellipsis symbol.
To show ellipsis on overflow:
- Add the overflow parameter.
- Assign TextOverflow.ellipsis to the overflow parameter of the TextField widget.
Code:
Row( children: const [ Expanded( child: Text( 'It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout.', style: TextStyle(fontSize: 58), softWrap: false, maxLines: 1, overflow: TextOverflow.ellipsis, // new ), ), ], )
Output:

Output after setting maxLines: 2 on Text Widget:
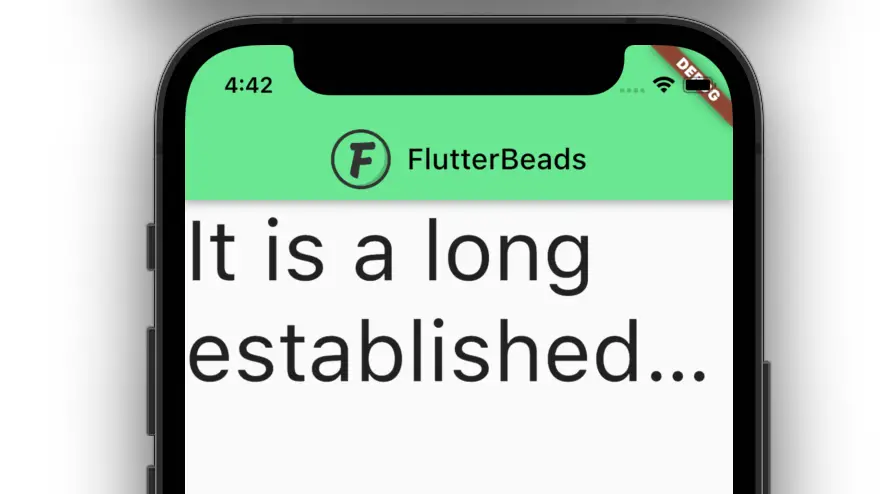
Fading the Text on Overflow
You can also represent the text continuity by fading the text. By default, it shows fading with white color. If the text is a single line it will fade the text from left to right. If it’s multi-line, fading will be shown from bottom to top.
To show fading on overflow:
- Add the overflow parameter.
- Assign TextOverflow.fade to the overflow parameter of the TextField widget.
Code:
Row( children: const [ Expanded( child: Text( 'It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout.', style: TextStyle(fontSize: 58), softWrap: false, maxLines: 1, overflow: TextOverflow.fade, //new ), ), ], )
Output:

Output after setting maxLines: 2 and softWrap: true on Text Widget:

Showing Full Text to Avoid Overflow
Sometimes you may want to display the full text on a screen by compromising the font size. To display the long text in a limited screen width, you can use FittedBox widget.
To show full text in a Row:
- Make sure your Text widget is inside the Row and an Expanded widget.
- Wrap your Text widget inside the FittedBox widget.
- Run the app.
Code:
Row( children: const [ Expanded( child: FittedBox( child: Text( 'It is a long established text.', style: TextStyle(fontSize: 58), softWrap: false, overflow: TextOverflow.ellipsis, ), ), ), ], )
Output:

Full Code
Here’s the full code:
import 'package:flutter/material.dart'; import 'wrapping_text/wrapping_text_demo.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: WrappingTextDemo(), ); } } class WrappingTextDemo extends StatelessWidget { const WrappingTextDemo({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return GestureDetector( onTap: () { FocusManager.instance.primaryFocus?.unfocus(); }, child: Scaffold( appBar: AppBar( centerTitle: true, title: Row( mainAxisAlignment: MainAxisAlignment.center, children: [ Image.asset( 'assets/images/logo.png', scale: 12, ), const SizedBox( width: 10, ), const Text( 'FlutterBeads', style: TextStyle(color: Colors.black), ), ], ), backgroundColor: const Color(0xff6ae792), ), body: Column( children: [ Row( children: const [ Expanded( child: Text( 'It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout.', style: TextStyle(fontSize: 58), softWrap: false, maxLines: 2, overflow: TextOverflow.ellipsis, ), ), ], ), Row( children: const [ Expanded( child: Text( 'It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout.', style: TextStyle(fontSize: 58), softWrap: true, maxLines: 2, overflow: TextOverflow.fade, ), ), ], ), Row( children: const [ Expanded( child: FittedBox( child: Text( 'It is a long established text.', style: TextStyle(fontSize: 58), softWrap: false, overflow: TextOverflow.ellipsis, ), ), ), ], ), ], ), ), ); } }
Thanks!
FAQs
How do you use text overflow ellipsis in Flutter?
To use text overflow ellipsis in Flutter, you can set the overflow
property of the Text
widget to TextOverflow.ellipsis
. This will display an ellipsis symbol at the end of the visible text when the text overflows the available space. Additionally, you can also set the maxLines
property to limit the number of visible lines before displaying the ellipsis symbol.
How do you break text to the next line in Flutter?
In Flutter, you can break text to the next line by setting the softWrap
property of the Text
widget to true
. By default, this property is set to false
, which means that long text will overflow the widget’s bounds. When softWrap
is true
, the text will break to the next line instead of overflowing.
Conclusion
In conclusion, text overflow can be a common problem faced by developers while creating beautiful UI designs for their Flutter apps. However, with the proven wrap strategies discussed in this tutorial, such as showing ellipsis and fading on overflowing text, developers can easily overcome this issue and create visually appealing and functional apps that provide a seamless user experience.
Would you like to check other interesting Flutter tutorials?
great article