A Date help users identify the time when the event has happened or will happen in the future. Displaying the date might be a critical feature for your app. For example, a messaging app must display a date on which the message is sent or received. But displaying the date without formatting might not be a good choice as the date might not be in the human-readable format. So in this tutorial, we’ll discover two ways to master DateTime format with DateFormat Flutter.
Note: The dates are formated and shown in the ‘en_US’ locale.
Bonus 🔥: You will get cheatsheet in this tutorial.
At the end of this tutorial, you’ll be able to format a DateTime in the following format and more:

Here’s what we’ll cover:
The Problem
The DateTime value generated in the app or received from somewhere else might not be readable to your users and might look something like this:

Ways to DateFormat or DateTime Format in Flutter
There are two ways you can format a DateTime in Flutter. You can use any of the following based on your requirement.
- Using Standard Formats
- Using Custom Pattern
1. Using Standard Formats
To DateFormat or DateTime Format in Flutter using a standard format, you need to use the intl
library and then use the named constructors from the DateFormat
class. Simply write the named constructor and then call the format()
method with providing the DateTime.
Step 1: Add the intl package.
dependencies: flutter: sdk: flutter # The following adds the Cupertino Icons font to your application. # Use with the CupertinoIcons class for iOS style icons. cupertino_icons: ^1.0.2 intl: ^0.17.0 # <-- SEE HERE
Step 2: Get the date that you want to format.
DateTime now = DateTime.now();
Step 3: Use the named constructor and then call the format() method and then provide the date.
String formattedDate = DateFormat.yMMMEd().format(now);
Note: The format function gives you the result in String.
Step 4: Use the formated string wherever you like.
print(formattedDate);
Code Example:
DateTime now = DateTime.now(); String formattedDate = DateFormat.yMMMEd().format(now); print(formattedDate);
Output:
Tue, Jan 25, 2022
Cheatsheet:
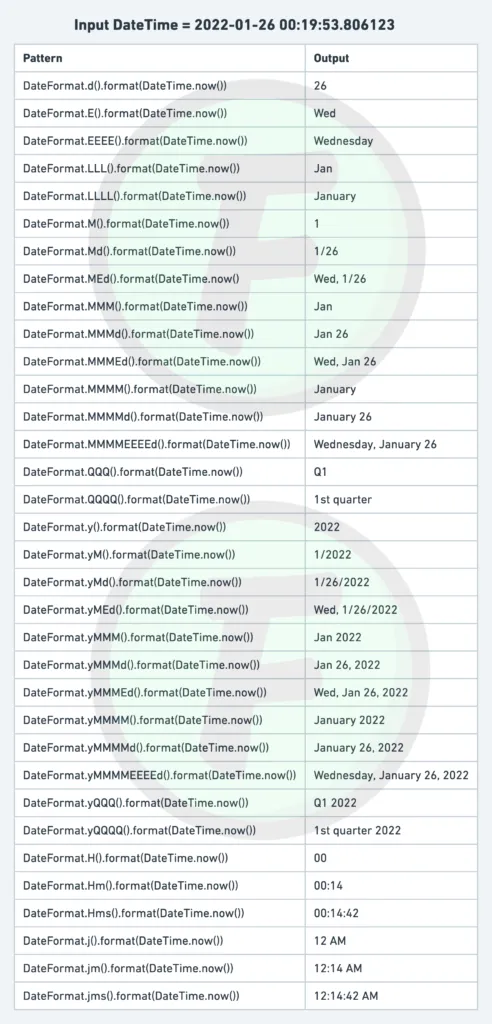
2. Using Custom Pattern
Sometimes, your designer may ask you to show a date in a format that is not part of the standard format. In such a situation, you can write your own customized pattern to format a date.
The steps to writing the custom pattern are almost the same as previous. The only change is, instead of adding the named constructor, you should write the exact pattern in which you want to format the date.
Code Example:
DateTime now = DateTime.now(); formattedDate = DateFormat('EEEE, MMM d, yyyy').format(now); print(formattedDate);
Output:
Tuesday, Jan 25, 2022
Cheatsheet:

Showing Locale based Date
Sometimes, you may want to format a date in a specific locale. For example, showing the date in Indian format.
To format the DateTime based on locale, you should first add the intl package as mentioned in the previous steps and then write the below code:
Code Example:
DateTime now = DateTime.now(); initializeDateFormatting('en-IN', '') .then((_) => print(DateFormat.yMd('en-IN').format(now)));
Output:
25/1/2022 <-- Date in Indian format
Conclusion
In this tutorial, we learned the 2 easy ways to DateFormat or DateTime Format in Flutter with practical examples. We also saw how you could display the Date based on locale, and with the help of a cheatsheet, you are now able to identify the pattern and format the DateTime accordingly.
FAQs
How to get current time in flutter?
To get the current time in Flutter, you can use the DateTime.now()
method. This method returns the current date and time as a DateTime
object. To get just the current time, you can use the TimeOfDay.now()
method, which returns a TimeOfDay
object representing the current time. Here’s an example:
DateTime now = DateTime.now(); TimeOfDay currentTime = TimeOfDay.now(); print('Current date and time: $now'); print('Current time: $currentTime'); //Output //Current date and time: 2023-04-04 15:23:45.678901 //Current time: 3:23 PM
How to get only date from datetime in flutter?
In Flutter, you can get only the date from DateTime by using the toLocal()
method to convert the DateTime object to the local time zone, and then using the toString()
method with a specified format string that only includes the date fields. Here’s an example:
DateTime now = DateTime.now(); String formattedDate = DateFormat('yyyy-MM-dd').format(now.toLocal());
How to get time in flutter?
To get the current time in Flutter, you can use the DateTime.now()
method. This will return a DateTime
object representing the current date and time. From this object, you can get the time using the hour
, minute
, and second
properties. Here is an example code snippet:
DateTime now = DateTime.now(); int hour = now.hour; int minute = now.minute; int second = now.second; print('$hour $minute $second');
How to convert datetime to string in flutter?
To convert a DateTime
object to a string in Flutter, you can use the toString()
method. By default, the toString()
method returns a string in the format “YYYY-MM-DD HH:MM:SS.mmmmmm”. If you need a different format, you can use the DateFormat
class. Here’s an example:
DateTime now = DateTime.now(); String formattedDate = DateFormat('yyyy-MM-dd – kk:mm').format(now); print(formattedDate);
Want to check some other awesome articles?